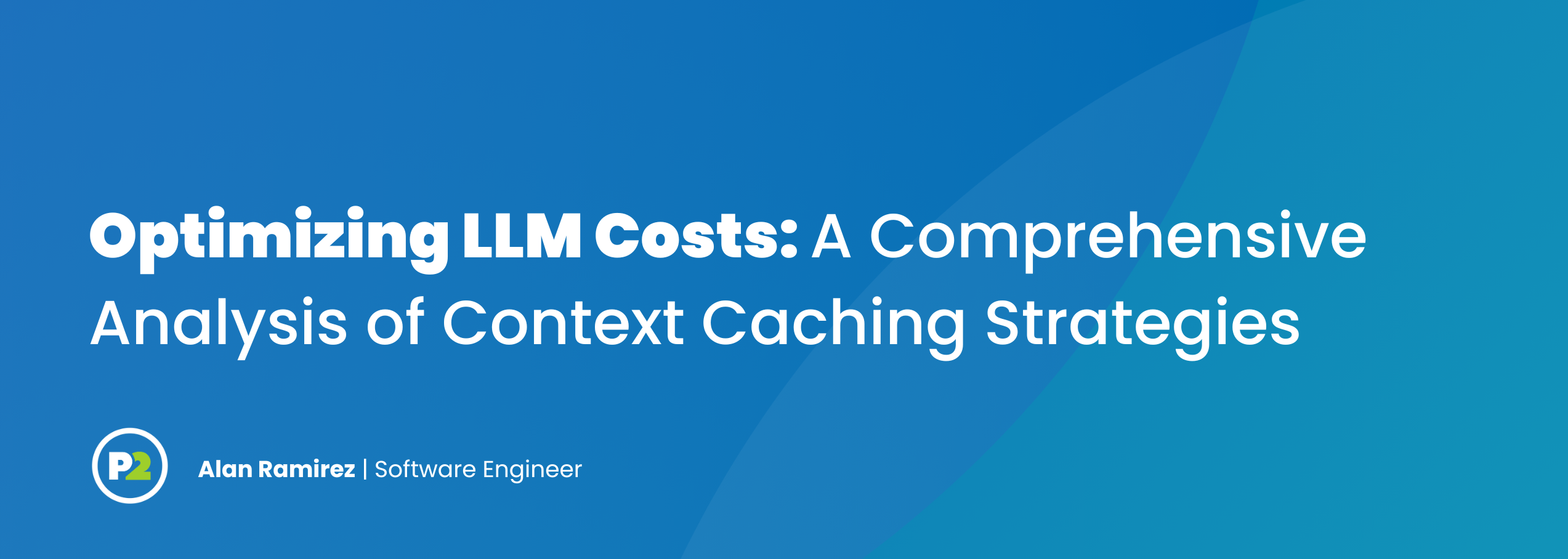
Optimizing LLM Costs: A Comprehensive Analysis of Context Caching Strategies
Introduction
Large Language Models (LLMs) have revolutionized how organizations process and generate natural language content, but their operational costs can become significant at scale. One of the most effective techniques for reducing these costs is context caching, which allows reuse of static prompt components across multiple requests. This article examines how the three major AI providers—Google (Gemini), Anthropic (Claude), and OpenAI—implement context caching, with detailed analysis of their technical approaches, pricing structures, and practical limitations.
The Technical Fundamentals of Context Caching
When interacting with LLMs, each request typically includes both static components (system instructions, guidelines, context) and dynamic content (user queries, new data). Context caching works by:
- Storing the static portions of prompts in memory
- Reusing these cached components in subsequent requests
- Only processing the new or changing elements
This approach significantly reduces token consumption and processing time for applications that send similar prompts repeatedly.
Provider-Specific Implementations
Google’s Gemini Context Caching
Google has implemented a cache-first approach that claims to reduce costs by approximately 75% for compatible workloads.
Technical Specifications:
- Compatible Models: Only stable versions of Gemini 1.5 Pro and Gemini 1.5 Flash
- Implementation Details: Requires specific version postfixes (e.g.,
gemini-1.5-pro-001
) - Minimum Cache Size: 32,768 tokens (a significant threshold)
- Cache Lifetime: Default 1 hour with customization options
Pricing Structure:
- Initial cache generation: Standard processing rates
- Cached content: Regular token prices plus maintenance fees
- Gemini 1.5 Flash: $0.01875 per million tokens (≤128k tokens) or $0.0375 (>128k tokens), plus $1 per million tokens per hour
- Gemini 2.0 Flash: $0.025 per million tokens for all content types plus $1 per million tokens per hour
API Implementation Example:
import google.generativeai as genai
import os
# Configure the API key
genai.configure(api_key=os.environ["GOOGLE_API_KEY"])
# Create model configuration with caching
model = genai.GenerativeModel(
model_name="gemini-1.5-pro-001",
cache_config={
"enabled": True,
"ttl_seconds": 3600 # 1 hour default
}
)
# Generate content using cache
response = model.generate_content(
"Your static prompt content here...",
)
# Access the cache ID for future reference
cache_id = response.cache_info.cache_id
# Subsequent request using the existing cache
cached_response = model.generate_content(
"Your dynamic content here...",
cache_id=cache_id
)
https://ai.google.dev/gemini-api/docs/pricing
https://ai.google.dev/gemini-api/docs/caching?lang=python
Anthropic’s Claude Prompt Caching
Anthropic takes a distinctive approach by implementing differential pricing for cache writes versus reads, optimizing for frequent reuse of cached content.
Technical Specifications:
- Minimum Requirements: Much lower thresholds at 1,024 tokens (Sonnet/Opus) or 2,048 tokens (Haiku)
- Cache Lifecycle: “Ephemeral” 5-minute lifetime, refreshed with each read operation
- Integration Support: Compatible with LangChain’s ChatAnthropic implementation
Pricing Structure:
- Cache writing: 25% premium over standard token pricing
- Cache reading: 90% discount compared to standard pricing
- Claude 3.7 Sonnet: $3.75/million tokens (writes), $0.30/million tokens (reads)
- Claude 3 Haiku: $0.25/million tokens (writes), $0.03/million tokens (reads)
- Claude 3 Opus: $18.75/million tokens (writes), $1.50/million tokens (reads)
API Implementation Example:
from anthropic import Anthropic
client = Anthropic()
# Initial request that creates the cache
response = client.messages.create(
model="claude-3-7-sonnet-20240229",
system="Static system prompt that will be cached",
messages=[
{"role": "user", "content": "Dynamic user content"}
],
cache_control={
"mode": "write", # Create a cache
"ttl": 300 # 5 minute lifetime
}
)
# The cache_id from the response
cache_id = response.usage.cache_id
# Subsequent request using the cache
cached_response = client.messages.create(
model="claude-3-7-sonnet-20240229",
cache_control={
"mode": "read", # Read from cache
"cache_id": cache_id # Use the previously created cache
},
messages=[
{"role": "user", "content": "New dynamic content"}
]
)
https://docs.anthropic.com/en/docs/build-with-claude/prompt-caching#what-is-the-cache-lifetime
OpenAI’s Automatic Prompt Caching
OpenAI has implemented a more transparent approach that activates automatically when conditions are met, requiring minimal developer intervention.
Technical Specifications:
- Eligible Models: gpt-4.5-preview, gpt-4o, gpt-4o-mini, o1-mini
- Minimum Cache Size: 1,024 tokens
- Activation: Automatic when token thresholds are met
- Cache Persistence: 5-10 minutes standard, up to one hour during off-peak periods
Pricing Impact:
- Claimed 50% cost reduction for eligible requests
- No additional configuration or pricing tiers
Implementation Note: Since OpenAI’s caching activates automatically, no special API code is required. However, structuring prompts with static content first maximizes cache utilization.
https://platform.openai.com/docs/guides/prompt-caching
Technical Architecture Considerations
Optimizing Prompt Structure
The effectiveness of context caching depends significantly on prompt architecture. For maximum efficiency across all providers:
- Front-load static content: Place unchanging system instructions, context, and guidelines at the beginning of prompts
- Isolate dynamic elements: Keep user-specific or changing content separated and minimal
- Batch similar requests: When possible, group requests that use the same static content
Architectural Diagram
┌─────────────────────────────────┐
│ Application Layer │
└───────────────┬─────────────────┘
│
┌───────────────▼─────────────────┐
│ Prompt Structure │
│ ┌─────────────────────────────┐ │
│ │ Static Content (Cached) │ │
│ ├─────────────────────────────┤ │
│ │ Dynamic Content (New) │ │
│ └─────────────────────────────┘ │
└───────────────┬─────────────────┘
│
┌───────────────▼─────────────────┐
│ Provider Cache Layer │
│ ┌─────────────┐ ┌─────────────┐ │
│ │ Cache Write │ │ Cache Read │ │
│ └─────────────┘ └─────────────┘ │
└───────────────┬─────────────────┘
│
┌───────────────▼─────────────────┐
│ LLM Processing │
└─────────────────────────────────┘
Comparative Analysis
Each provider’s implementation offers distinct advantages and limitations worth considering when designing LLM-powered applications:
Provider | Min. Tokens | Lifetime | Cost Reduction | Best Use Case |
Gemini | 32,768 | 1 hour | ~75% | Large, consistent workloads |
Claude | 1,024/2,048 | 5 min (refresh) | ~90% for reads | Frequent reuse of medium prompts |
OpenAI | 1,024 | 5-60 min | ~50% | General-purpose applications |
Technical Limitations
Gemini’s approach provides excellent persistence but the 32K token minimum excludes many common use cases, limiting its utility to applications with substantial prompt sizes. This high threshold means smaller applications cannot benefit from caching.
Claude’s implementation offers the most flexible token thresholds but requires careful attention to timing. The 5-minute cache lifetime could lead to frequent recaching if operations have gaps, potentially increasing costs for intermittent workloads.
OpenAI’s solution provides the most transparent implementation but offers less control over cache behavior. Since it activates automatically, developers may already be benefiting without realizing it, potentially reducing opportunities for further optimization.
Real-World Implementation Strategies
Based on our analysis, we recommend the following technical approaches for different application patterns:
For High-Volume Applications with Large, Consistent Prompts
- Recommended Provider: Gemini
- Implementation Strategy:
- Structure large system prompts and context as cacheable components
- Maintain regular request cadence to maximize cache utilization
- Consider batching similar requests to optimize the hourly cache fee
For Applications with Frequent, Smaller Requests
- Recommended Provider: Claude
- Implementation Strategy:
- Design for continuous cache refreshing through regular activity
- Implement request scheduling to prevent cache expiration
- Accept the higher write costs in exchange for significantly reduced read costs
For General-Purpose or Mixed Workloads
- Recommended Provider: OpenAI
- Implementation Strategy:
- Structure prompts for automatic cache optimization
- No special implementation required beyond prompt architecture
Conclusion
Context caching represents a significant advancement in making LLM deployments more cost-effective at scale. The optimal implementation depends on your specific application patterns, request volumes, and prompt structures.
For most applications, the ideal approach involves:
- Structuring prompts with caching in mind (static content first)
- Selecting a provider based on your token volume and request patterns
- Implementing appropriate cache lifecycle management
- Continuously monitoring usage patterns to refine your approach
As these technologies mature, we can expect more sophisticated caching mechanisms that further optimize the cost-performance balance of LLM applications. Organizations that master these techniques now will be well-positioned to scale their AI applications efficiently as language models continue to evolve.