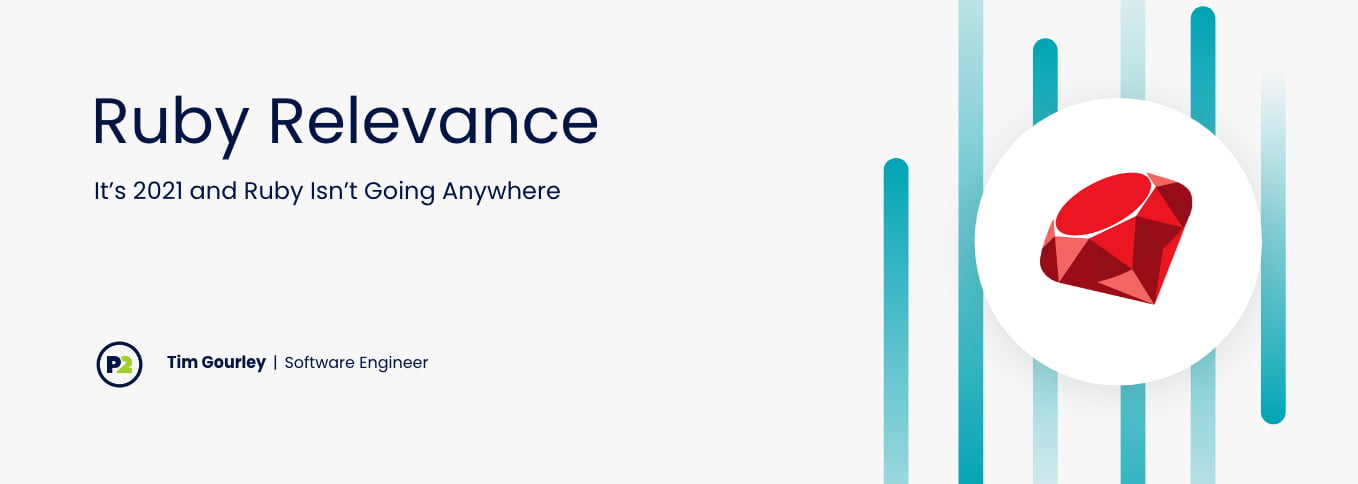
Ruby Relevance: It’s 2021 and Ruby Isn’t Going Anywhere
Choosing the right language and web framework can be difficult when deciding on a tech stack for an upcoming project. Balancing maintainability, scalability, programmer happiness, and speed of development can seem like a house of cards destined to fail. There are many languages and frameworks from which to choose. Ruby is a superb choice for a tech stack, despite having its trials over the years. When looking to develop, iterate rapidly, and run your application, Ruby and Ruby on Rails are contenders to consider.
Community
The communities behind the Ruby programming language and the Ruby on Rails web framework maintain a high level of activity. There are more than 4,000 Rails contributors to the code base on GitHub with active changes and regular releases.
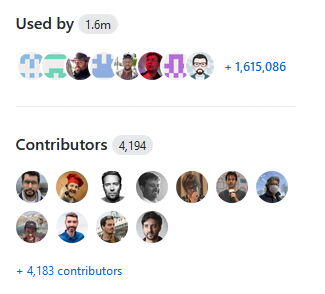
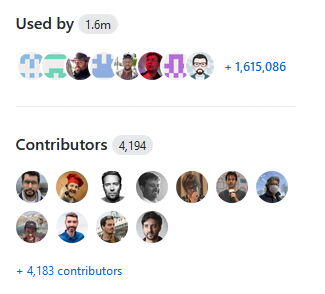
Ruby has a more focused core of dedicated contributors. However, they still release frequently and continue to improve the language and its performance.
There are over 165,000 Ruby gems published, with many of them maintained. What’s a gem? It’s a pre-packaged library of code that can be used in your own applications. As a result, removing the headaches of writing boilerplate code for a variety of activities.
The people in the community are genuine and helpful. You can meet and interact with them at local user groups or even at annual conferences. For instance, Rubyconf and Railsconf are great ways to interact with many developers in the community. Additionally, you can keep abreast of the latest and greatest, as well as user groups.
New Features
Ruby’s annual Christmas release this past year brought Ruby 3.0, which is dubbed “Ruby 3×3“. The goal of the release was to focus on performance, concurrency, and typing. Also, to make Ruby 3 times faster than Ruby 2.
Ruby 3 Static Typing
Ruby 3 includes tools for static typing of your code. First, a descriptor language called RBS describes the structure of your ruby code. Then, there’s a corresponding type checker tool named Steep can check your code against the RBS definitions. Therefore, development is quicker, as bugs are easier to detect and code compiles faster.
# automobile.rb -- The file contains your code
class Automobile
attr_accessor :name
def initialize(name)
@name = name
end
end
class SportUtilityVehicle < Automobile
attr_accessor :hatch
def initialize(name:, hatch: true)
super(name)
@hatch = hatch
end
def hatchback?
@hatch
end
end
Automobile.new("Subaru Impreza")
SportUtilityVehicle.new(name: "Subaru Forester", hatch: true)
# automobile.rbs -- This file describes the types for your code
class Automobile
attr_accessor name: String
def initialize: (String name) -> String
end
class SportUtilityVehicle < Automobile
@name: String
attr_accessor hatch: true
def initialize: (name: String, ?hatch: true) -> true
def hatchback?: -> true
end
One-Line Pattern Matching
Additionally, recent versions added pattern matching, similar to what you’d see in other functional languages like Elixir. Ruby 3 redesigned and simplified the process, using the =>
character to perform a rightward assignment:
{ name: 'Tim', role: 'Developer', company: 'Phase 2' } => {name:}
puts "I matched the name '#{name}'"
# I matched the name 'Tim'
New Features in Rails 6
Progress on Ruby on Rails has been marching forward as well, with the release of Rails 6 and 6.1. Therefore, the codebase is maturing. By switching frontend management to use Webpacker by default, Rails works with modern front-end JavaScript frameworks. Additionally, it aligns with advancements in the JavaScript language itself. Extra features are being added to Rails as well. These include the rich text editor with Action Text and a straightforward way to send email with Action Mailbox. Also, parallel testing in Rails allows for fast concurrent tests. Developers can use more complex database structures with per-database connection switching and horizontal scaling.
Performance Improvements
To help with performance, Ruby switched to MJIT, a “method-based just in time” compiler. It compiles often-used instructions into binary code, which then compiles into YARV instructions that the Ruby Virtual Machine reads.
Additionally, for concurrency, they implemented “ractors”, an actor-model-like concurrent abstraction allowing for parallel execution without thread-safety concerns. These have often bogged down Ruby in the past. While this feature is marked as “experimental”, it shows promise. Threaded operations saw significant performance increase when running with parallel actors.
Ruby 3 also added the “Fiber Scheduler” for “intercepting blocking operations”. This adds hooks to existing concurrency calls that use fibers on the backend. These increase the performance of existing code with no code changes needed on the developer’s part. For example, RubyKaigi has a video getting into the nuts and bolts of how it works.
Jobs
Starting in 2005, organizations embraced Ruby and Rails as the de facto way to create their products for the web. These organizations ranged from established enterprises to smaller startups. Twitter, GitHub, Bleacher Report, Shopify, Etsy, Hulu, Twitch, and many others grew their brands by relying on Ruby on Rails. Companies were able to focus more on their product and business without the stress of writing massive amounts of code.
Some companies pivoted away from using Ruby as their primary language or framework. Most cited performance issues when dealing with massive amounts of traffic. However, most of the companies listed above still use Ruby as part of their tech stack. Stackshare shows that almost 5,000 of registered companies use it as part of their tech stack. A LinkedIn search shows thousands of jobs in the USA for Ruby on Rails developers.
High demand exists and will for the foreseeable future, so Ruby isn’t going anywhere. There will continue to be new Ruby projects and ongoing maintenance for existing systems. Job security exists for a developer with Ruby in the tool belt.
Developer Happiness
Yukihiro “Matz” Matsumoto had the idea to create his own programming language. The goal was to follow the “principle of least astonishment“, to meet developer expectations and behave logically. What he ended up with was Ruby — and it is incredibly expressive.
For instance, here are many ways of accomplishing a task in Ruby. It provides an elegant set of tools and building blocks and lets the developer mold them with minimal constraints. It brings a sense of artistry into development that several languages do not allow (or even actively discourage). Similarly, consider the Fibonacci Sequence, a series of numbers where the next number in the sequence is the sum of the previous two. With Ruby, you can take advantage of features like parallel assignment to craft a readable, simple-to-understand implementation:
def fibonacci(number)
item1, item2 = [0, 1]
(number).times do
item1, item2 = item2, item1 + item2
end
item1
end
10.times do |item|
puts fibonacci(item)
end
# Output:
# 0
# 1
# 1
# 2
# 3
# 5
# 8
# 13
# 21
# 34
This example can be written significantly shorter using recursion and the ternary operator. See how concise and flexible the language is at its core:
def fibonacci(number)
number < 2 ? number : fibonacci(number - 1) + fibonacci(number - 2)
end
In other words, all of that beauty and freedom leads to a pleasant development experience, creating developer happiness. How many developers maintaining ancient COBOL code do what they do with a smile? Likely not nearly as many developers writing and maintaining Ruby code.
The Takeaway
In conclusion, with its maturity and thriving community, choosing a Ruby-based tech stack is a valid choice. Using Ruby may significantly increase productivity, developer happiness and could lead to shorter development cycles.
However, a team must consider the use case for your particular problem when choosing any part of a tech stack. For example, if you generate high traffic like Twitter or perform complex big data analysis, Ruby might not be the best choice for you. It’s always best to use the right tool for the right job. For the average audience needing to iterate rapidly over development, Ruby is a splendid choice in 2021.